Ryzen AI 1.3がリリースされていたので、環境構築とテストを行う。
Ryzen AI 1.2 から 1.3 へのアップデート概要
- Quark量子化器の最初のリリース
- 混合精度データ型のサポート
- Copilot+アプリケーションとの互換性
※前回の記事はこちら: RyzenAI 1.2 インストール手順
Ryzen AI NPUドライバーのインストール手順
既存ドライバーのアンインストール
Windowsの「デバイスマネージャー」から既存のNPUドライバーを削除する。再起動してください。
新しいNPUドライバーの導入
- AMD公式サイトから
npu_sw_installer.exe
をダウンロード - 管理者権限で実行
- 再起動
- Neural processorsが表示されていればドライバのインストールは完了
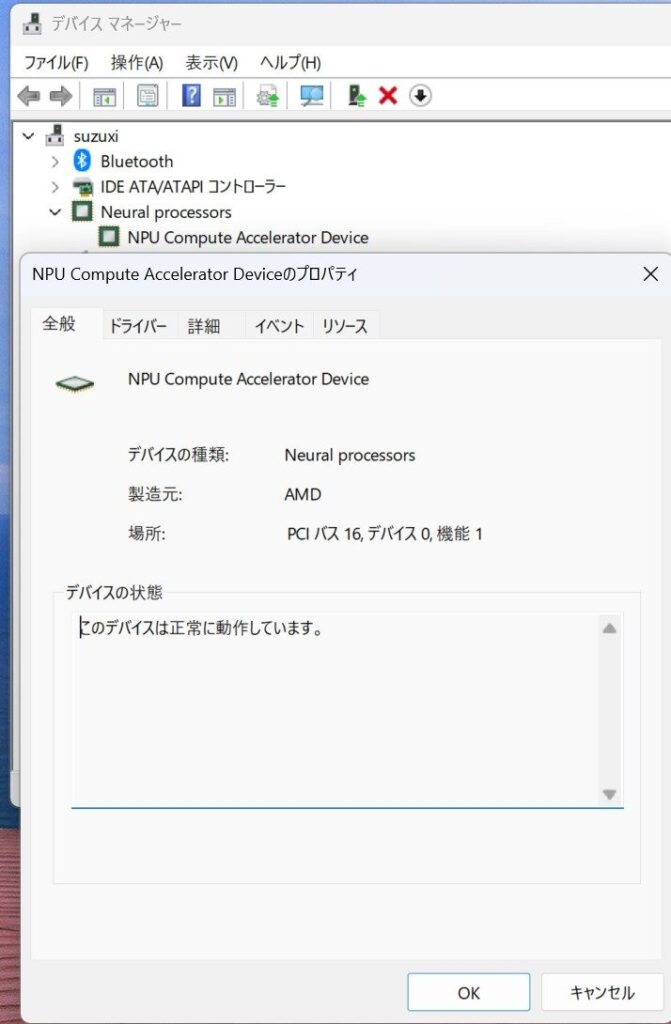
RyzenAIの環境をインストール
- ryzen-ai-rt-1.3.0-20241204.msiをダウンロード&実行
- Anacondaにryzen-ai-1.3.0の環境が作成される
- AnacondaのTerminalでryzen-ai-1.3.0を立ち上げる
QUICKテスト実行時のUnicodeDecodeError対策
Uコマンドプロンプトで以下を実行してからスクリプトを起動してください。
前の記事と同じように変更して実行するエラーになった。
chcp 65001
コードを以下にように変更した。
変更箇所は文字コードになる
if "PCI\\VEN_1022&DEV_1502&REV_00" in stdout.decode("latin1"):
apu_type = "PHX/HPT"
if "PCI\\VEN_1022&DEV_17F0&REV_00" in stdout.decode("latin1"):
apu_type = "STX"
if "PCI\\VEN_1022&DEV_17F0&REV_10" in stdout.decode("latin1"):
apu_type = "STX"
if "PCI\\VEN_1022&DEV_17F0&REV_11" in stdout.decode("latin1"):
apu_type = "STX"
if "PCI\\VEN_1022&DEV_17F0&REV_20" in stdout.decode("latin1"):
apu_type = "KRK"
return apu_type
テストモデルの実行と対策
文字コードの対策はしたが今度は、モデルパスが指定できていないことでエラーが発生した。モデルパスを直接指定を行った。
try:
model_path = os.path.join(
os.environ["RYZEN_AI_INSTALLATION_PATH"],
"quicktest",
"test_model.onnx",
)
print(f"Model path: {model_path}")
sess_options = ort.SessionOptions()
sess_options.graph_optimization_level = (
ort.GraphOptimizationLevel.ORT_ENABLE_EXTENDED
)
session = ort.InferenceSession(model_path, sess_options)
print("InferenceSession created successfully")
except Exception as e:
print(f"Failed to create InferenceSession: {e}")
(ryzen-ai-1.3.0) PS C:\Program Files\RyzenAI\1.3.0\quicktest> python .\quicktest.py
Detected APU type: PHX/HPT
Setting environment for PHX/HPT
Firmware path: C:\Program Files\RyzenAI\1.3.0\voe-4.0-win_amd64\xclbins\phoenix\1x4.xclbin
Model path: C:\Program Files\RyzenAI\1.3.0\quicktest\test_model.onnx
InferenceSession created successfully
Test Passed
コード全体
# Copyright (C) 2023 Advanced Micro Devices, Inc. All rights reserved.
import os
import sys
import subprocess
import numpy as np
import onnxruntime as ort
def get_apu_info():
# Run pnputil as a subprocess to enumerate PCI devices
command = r"pnputil /enum-devices /bus PCI /deviceids "
process = subprocess.Popen(
command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE
)
stdout, stderr = process.communicate()
# Check for supported Hardware IDs
apu_type = ""
if "PCI\\VEN_1022&DEV_1502&REV_00" in stdout.decode("latin1"):
apu_type = "PHX/HPT"
if "PCI\\VEN_1022&DEV_17F0&REV_00" in stdout.decode("latin1"):
apu_type = "STX"
if "PCI\\VEN_1022&DEV_17F0&REV_10" in stdout.decode("latin1"):
apu_type = "STX"
if "PCI\\VEN_1022&DEV_17F0&REV_11" in stdout.decode("latin1"):
apu_type = "STX"
if "PCI\\VEN_1022&DEV_17F0&REV_20" in stdout.decode("latin1"):
apu_type = "KRK"
return apu_type
def set_environment_variable(apu_type):
install_dir = os.environ["RYZEN_AI_INSTALLATION_PATH"]
match apu_type:
case "PHX/HPT":
print("Setting environment for PHX/HPT")
firmware_path = os.path.join(
install_dir, "voe-4.0-win_amd64", "xclbins", "phoenix", "1x4.xclbin"
)
print(f"Firmware path: {firmware_path}")
os.environ["XLNX_VART_FIRMWARE"] = firmware_path
os.environ["NUM_OF_DPU_RUNNERS"] = "1"
os.environ["XLNX_TARGET_NAME"] = "AMD_AIE2_Nx4_Overlay"
case "STX" | "KRK":
print("Setting environment for STX")
firmware_path = os.path.join(
install_dir,
"voe-4.0-win_amd64",
"xclbins",
"strix",
"AMD_AIE2P_Nx4_Overlay.xclbin",
)
print(f"Firmware path: {firmware_path}")
os.environ["XLNX_VART_FIRMWARE"] = firmware_path
os.environ["NUM_OF_DPU_RUNNERS"] = "1"
# デバッグ用の出力を追加
apu_type = get_apu_info()
print(f"Detected APU type: {apu_type}")
set_environment_variable(apu_type)
# ONNX Runtimeの初期化部分を確認
try:
model_path = os.path.join(
os.environ["RYZEN_AI_INSTALLATION_PATH"],
"quicktest",
"test_model.onnx",
)
print(f"Model path: {model_path}")
sess_options = ort.SessionOptions()
sess_options.graph_optimization_level = (
ort.GraphOptimizationLevel.ORT_ENABLE_EXTENDED
)
session = ort.InferenceSession(model_path, sess_options)
print("InferenceSession created successfully")
except Exception as e:
print(f"Failed to create InferenceSession: {e}")
def preprocess_random_image():
image_array = np.random.rand(3, 32, 32).astype(np.float32)
return np.expand_dims(image_array, axis=0)
# inference on random image data
input_data = preprocess_random_image()
try:
outputs = session.run(None, {"input": input_data})
except Exception as e:
print(f"Failed to run the InferenceSession: {e}")
sys.exit(1) # Exit the program with a non-zero status to indicate an error
else:
print("Test Passed")
関連記事: Ryzen AI 1.3 リリース記事